This tutorial series focuses on core Java – “Back to Basics”. We’re going to cover Java core concepts, collections, streams, IO and more.
本系列教程的重点是核心Java – “Back to Basics”。我们将介绍Java的核心概念、集合、流、IO等内容。
If you are new to Java, this series will go over the basic syntax of the language, introduce classes and objects and a few simple examples of using common Java structures.
如果你是Java的新手,这个系列将讲述语言的基本语法,介绍类和对象,以及使用常见Java结构的一些简单例子。
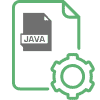
2. Core Java Examples
2.核心Java实例
In this section, we’ll continue with more Core Java examples that show how to use the basic concepts of the language.
在本节中,我们将继续介绍更多的Core Java例子,展示如何使用该语言的基本概念。
-
Comparing Objects in Java -
Wrapper Classes in Java -
Quick Guide to java.lang.System -
Object Type Casting in Java -
Java 8 – Powerful Comparison with Lambdas -
Guide To Java 8 Optional(popular) -
Guide to UUID in Java -
The StackOverflowError in Java -
The “final” Keyword in Java -
Immutable Objects in Java -
Anonymous Classes in Java -
Command-Line Arguments in Java -
Java toString() Method -
Java 14 Record Keyword -
Iterating over Enum Values in Java -
Comparing Dates in Java -
RegEx for matching Date Pattern in Java -
Period and Duration in Java -
Java Timer -
Number Formatting in Java -
How to Round a Number to N Decimal Places in Java -
Java – Random Long, Float, Integer and Double -
Comparing Long Values in Java -
Convert Date to LocalDate or LocalDateTime and Back(popular) -
Using an Interface vs. Abstract Class in Java
In this series, we’ll learn how to work with Strings with examples of common operations and conversions.
在这个系列中,我们将通过常见操作和转换的例子来学习如何使用字符串。
Concurrency is a large area in Java, but it’s also an important topic to understand. In this series, we’ll go over the core concepts and learn how to work with threads using practical examples.
并发是Java中的一个大领域,但它也是一个需要理解的重要话题。在这个系列中,我们将复习核心概念,并通过实际例子学习如何使用线程。
This tutorials will go over the main data structures in Java and common operations we can perform with them.
本教程将介绍Java中的主要数据结构以及我们可以用它们进行的常见操作。
This series is a comprehensive guide to working with the Stream API introduced in Java 8.
本系列是关于使用Java 8中引入的Stream API的全面指南。
This series cover common I/O operations in Java, including working with files, Readers and Input/OuputStreams.
这个系列涵盖了Java中常见的I/O操作,包括与文件、读取器和Input/OuputStreams一起工作。
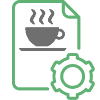
8. Advanced Java Examples
8.高级Java实例
Let’s have a look at more advanced use cases with Java.
让我们来看看Java的更多高级用例。
-
Composition, Aggregation, and Association in Java -
Class Loaders in Java -
A Guide to Java 9 Modularity -
Guide to Escaping Characters in Java RegExps -
Introduction to Java Serialization -
Practical Java Examples of the Big O Notation -
Stack Memory and Heap Space in Java -
Setting the Java Version in Maven (popular) -
Hashing a Password in Java -
Different Ways to Capture Java Heap Dumps -
Understanding Memory Leaks in Java -
How to Replace Many if Statements in Java -
Java equals() and hashCode() Contracts -
Guide to System.gc() -
A Guide to System.exit() -
Adding Shutdown Hooks for JVM Applications -
Do a Simple HTTP Request in Java(popular)
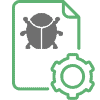
9. Tracking Java Development
9.追踪Java开发
-
New Features in Java 8 -
New Features in Java 9 -
Best Java Sites -
The State of Java in 2018 -
New Features in Java 10 -
The State of Java in 2019 -
New Features in Java 11 -
New Features in Java 12 -
New Features in Java 13 -
New Features in Java 14 -
What’s New in Java 15 -
New Features in Java 16 -
New Features in Java 17