Building a REST API is not a trivial task – from the high level RESTful constraints down to the the nitty-gritty of making everything work and work well.
构建REST API并不是一件简单的事情–从高水平的RESTful约束到使一切工作顺利进行的细枝末节。
Spring has made REST a first class citizen and the platform has been maturing in leaps and bounds. With the Spring 5 release, REST is now battle hardened and fully mature.
Spring使REST成为一流的公民,该平台一直在飞跃式地发展。随着Spring 5的发布,REST现在已经是战斗力强、完全成熟了。
With this guide, my aim is to organize the mountains of information that are available on the subject and guide you through properly building an API.
通过这本指南,我的目的是整理关于这个问题的大量信息,并指导你正确地构建一个API。
The guide starts with the basics – bootstrapping the REST API, the Spring MVC Configuration, basic customization.
本指南从基础知识开始 – 引导REST API,Spring MVC配置,基本的定制。
It then dives into the more advanced areas of REST – HATEOAS and pagination, Error Handling and testing.
然后,它深入到REST的更多高级领域 – HATEOAS和分页,错误处理和测试。
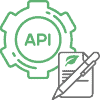
REST API Basics
REST API基础知识
-
Bootstrapping a Web Application -
Building a REST API -
The Spring @Controller and @RestController Annotations -
Error Handling for REST (popular) -
Entity To DTO Conversion for a Spring REST API -
Spring’s RequestBody and ResponseBody Annotations (popular) -
How to Read HTTP Headers in Spring REST Controllers -
Using Spring @ResponseStatus to Set HTTP Status Code -
Using Spring ResponseEntity to Manipulate the HTTP Response (popular)
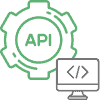
REST API Advanced Topics
REST API高级主题
-
Setting Up Swagger 2 with a Spring REST API(popular) -
Documenting a Spring REST API Using OpenAPI 3.0 (popular) -
Versioning a REST API -
REST Pagination -
ETags for REST -
Spring MVC Content Negotiation -
Spring REST API with Protocol Buffers -
A Custom Media Type for a Spring REST API -
Handling URL Encoded Form Data in Spring REST -
Generate Spring Boot REST Client with Swagger -
Spring REST API + OAuth2 + Angular -
Setting a Request Timeout for a Spring REST API
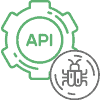
Test and Consume the API
测试和使用API
-
Test a REST API with Java -
The Guide to RestTemplate -
RestTemplate Post Request with JSON -
RestTemplate with Basic Authentication -
Get and Post Lists of Objects with RestTemplate -
Configure a RestTemplate with RestTemplateBuilder -
Spring RestTemplate Error Handling -
Uploading MultipartFile with Spring RestTemplate -
Spring WebClient vs. RestTemplate -
Spring WebClient Requests with Parameters -
Spring WebClient Filters -
Spring WebClient and OAuth2 Support -
Spring RestTemplate Request/Response Logging -
Reading an HTTP Response Body as a String in Java
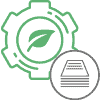
Other Spring Tutorials
其他Spring教程
-
Persistence with Spring Tutorial
Building the Persistence Layer of an application with Spring and Hibernate, JPA, Spring Data, etc -
Spring Exceptions Tutorial
Common Exceptions in Spring with examples – why they occur and how to solve them quickly -
Security with Spring
How to Secure an MVC project, Login and Logout, how to Secure a REST Service, Basic and Digest Authentication with Spring Security